Function GraSetAttrArea() Foundation
Determines attributes for areas which are drawn by GraArc() or GraBox()
GraSetAttrArea( [<oPS>], [<aAttributes>] ) --> aOldAttributes
Array element | #define | value | Default value |
---|---|---|
GRA_AA_COLOR | GRA_CLR_* | GRA_CLR_NEUTRAL |
GRA_AA_BACKCOLOR | GRA_CLR_* | GRA_CLR_BACKGROUND |
GRA_AA_MIXMODE | GRA_FGMIX_* | GRA_FGMIX_OVERPAINT |
GRA_AA_BGMIXMODE | GRA_BGMIX_* | GRA_BGMIX_LEAVEALONE |
GRA_AA_SYMBOL | GRA_SYM_* | GRA_SYM_SOLID |
The function GraSetAttrArea() returns a copy of an array containing the currently set area attributes. When new attributes are specified by <aAttributes>, the function returns an array with the previous attributes.
The function GraSetAttrArea() determines the attributes for areas. It has an effect when graphic primitives such as GraArc() and GraBox() are used to draw a filled area. The area attributes are also used by the function GraPathFill(), which fills a graphic path. The area attributes which can be specified are as follows:
Array element | Description |
---|---|
GRA_AA_COLOR | Foreground color |
GRA_AA_BACKCOLOR | Background color |
GRA_AA_MIXMODE | Color mix attribute for foreground |
GRA_AA_BGMIXMODE | Color mix attribute for background |
GRA_AA_SYMBOL | Fill pattern |
All area attributes remain until the function GraSetAttrArea() is called again. The exceptions to this are the colors, which are redefined with GraSetColor().
Foreground and background color
By default the colors used are those set by GraSetColor(). Separate foreground and background color can be specified for areas. If the area is to have a border, the color for the border is specified by the function GraSetAttrLine().
Mix attributes
Mix attributes for colors are significant when areas overlap. If area A cuts into area B, the color of the common intersect area is influenced by the mix attribute. Important mix attributes for the foreground color are GRA_FGMIX_OVERPAINT (area A overpaints area B) and GRA_FGMIX_OR (the common intersect area receives another color)
Another important mix attribute is GRA_FGMIX_XOR. This attribute effectively deletes a graphic primitive from the screen when it is executed or displayed a second time at the same coordinates (a detailed description of the mix attributes is found in chapter "The Xbase++ Graphics Engine (GRA)"of the Xbase++ documentation).
Fill pattern
The following illustration shows the fill patterns which can be selected by GraSetAttrArea(). Some of them are provided slightly different by the OS/2 and Windows platforms, respectively.
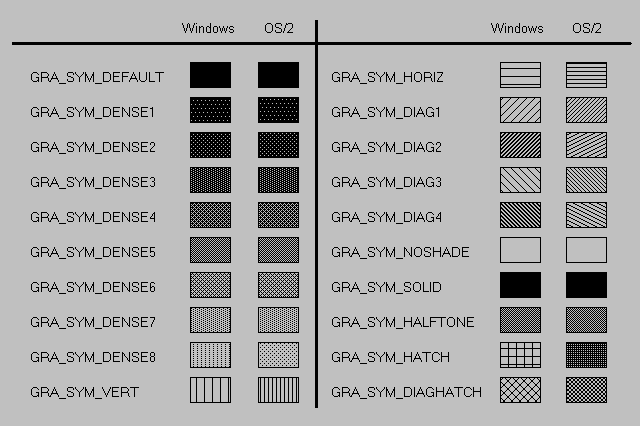
// This example displays all available fill patterns for areas.
#include "Gra.ch"
PROCEDURE Main
LOCAL oFont, aAttr, aSymbol, i, nX := 20, nY := 390, nCount
SetColor("N/W") // fill window with pale gray
CLS
oFont := XbpFont():New() // determine font
oFont:create("12.System VIO")
GraSetFont( NIL, oFont )
// all #define constants
aSymbol:= { ; // as text and as a value
{ "GRA_SYM_DEFAULT ", GRA_SYM_DEFAULT }, ;
{ "GRA_SYM_DENSE1 ", GRA_SYM_DENSE1 }, ;
{ "GRA_SYM_DENSE2 ", GRA_SYM_DENSE2 }, ;
{ "GRA_SYM_DENSE3 ", GRA_SYM_DENSE3 }, ;
{ "GRA_SYM_DENSE4 ", GRA_SYM_DENSE4 }, ;
{ "GRA_SYM_DENSE5 ", GRA_SYM_DENSE5 }, ;
{ "GRA_SYM_DENSE6 ", GRA_SYM_DENSE6 }, ;
{ "GRA_SYM_DENSE7 ", GRA_SYM_DENSE7 }, ;
{ "GRA_SYM_DENSE8 ", GRA_SYM_DENSE8 }, ;
{ "GRA_SYM_VERT ", GRA_SYM_VERT }, ;
{ "GRA_SYM_HORIZ ", GRA_SYM_HORIZ }, ;
{ "GRA_SYM_DIAG1 ", GRA_SYM_DIAG1 }, ;
{ "GRA_SYM_DIAG2 ", GRA_SYM_DIAG2 }, ;
{ "GRA_SYM_DIAG3 ", GRA_SYM_DIAG3 }, ;
{ "GRA_SYM_DIAG4 ", GRA_SYM_DIAG4 }, ;
{ "GRA_SYM_NOSHADE ", GRA_SYM_NOSHADE }, ;
{ "GRA_SYM_SOLID ", GRA_SYM_SOLID }, ;
{ "GRA_SYM_HALFTONE ", GRA_SYM_HALFTONE }, ;
{ "GRA_SYM_HATCH ", GRA_SYM_HATCH }, ;
{ "GRA_SYM_DIAGHATCH", GRA_SYM_DIAGHATCH } ;
}
aAttr := Array( GRA_AA_COUNT ) // create array for
nCount := 1 // area attributes
FOR i:=1 TO LEN( aSymbol )
aAttr[ GRA_AA_SYMBOL ] := aSymbol[i,2]
GraSetAttrArea( NIL, aAttr ) // set area attributes
// output characters
GraStringAt( NIL, { nX+ 10, nY-35*nCount }, aSymbol[i,1] )
// draw filled box
GraBox( NIL, { nX+170, nY-35*nCount- 5 }, ;
{ nX+250, nY-35*nCount+20 }, ;
GRA_OUTLINEFILL )
nCount++
IF i == 10
nX += 310
nCount := 1
ENDIF
NEXT
Inkey(0) // wait for key press
RETURN
// In the example the result of the mix attribute GRA_FGMIX_XOR
// is demonstrated while a filled circle is drawn which follows
// the mouse pointer. When the mouse pointer moves, the circle
// is redrawn at the previous position which deletes it from
// the screen.
#include "Gra.ch"
#include "Appevent.ch"
PROCEDURE Main
LOCAL nX := 250, nY := 150, aAttr, mp1, mp2, nEvent := 0
SetColor("N/W") // fill window with pale gray
CLS
SetMouse( .T. ) // activate mouse
SetAppWindow():mouseMode := 2 // graphic coordinates
SetAppWindow():autoMark := .F. // turn off marking
aAttr := Array( GRA_AA_COUNT ) // area attributes
aAttr [ GRA_AA_COLOR ] := GRA_CLR_RED
aAttr [ GRA_AA_SYMBOL ] := GRA_SYM_DIAG4
aAttr [ GRA_AA_MIXMODE ] := GRA_FGMIX_XOR
graSetAttrArea( NIL, aAttr )
aAttr := Array( GRA_AL_COUNT ) // line attributes
aAttr [ GRA_AL_COLOR ] := GRA_CLR_DARKBLUE
aAttr [ GRA_AL_MIXMODE ] := GRA_FGMIX_XOR
graSetAttrLine( NIL, aAttr )
// first draw circle
GraArc( NIL, { nX, nY }, 30, , , , GRA_OUTLINEFILL )
DO WHILE nEvent <> xbeM_RbClick // terminate with
// right mouse click
nEvent := AppEvent( @mp1, @mp2,,0) // retrieve event
IF Valtype(mp1) == "A" .AND. ;
( mp1[1] <> nX .OR. mp1[1] <> nY ) .AND. ;
NextAppEvent() == xbe_None // mouse pointer has
// new position
// delete circle
GraArc( NIL, { nX, nY }, 30,,,, GRA_OUTLINEFILL )
nX := mp1[1] // keep mouse position
nY := mp1[2] // redraw circle
GraArc ( NIL, { nX, nY }, 30,,,, GRA_OUTLINEFILL )
ENDIF
ENDDO
RETURN
If you see anything in the documentation that is not correct, does not match your experience with the particular feature or requires further clarification, please use this form to report a documentation issue.