Attributes for graphic primitives Foundation
Attributes are set before drawing in order to modify the output of graphic primitives. These settings are valid until they are reset. For example, colors, line types and fill patterns for areas can be defined. The number of attributes differs for different graphic primitives. Attributes are defined in the form of an attribute array whose size and elements are determined using constants defined in the GRA.CH file. Also, the values that are entered in the elements of the attribute array are represented using #define constants. The basic procedure for changing attributes of graphic primitives is shown in the following code:
aAttribute := Array( GRA_AL_COUNT ) // create empty attribute
// array for lines
aAttribute[ GRA_AL_TYPE ] := GRA_LINETYPE_SHORTDASH
aAttribute[ GRA_AL_COLOR ] := GRA_CLR_RED // select line type "short
// dash" and the color red
GraSetAttrLine( , aAttribute ) // set line attributes
This code illustrates the naming convention used by the GRA engine for the #define constants. All the constants begin with the prefix GRA_. Generally another prefix is also included to identify the group of GRA_ constants and a suffix is included at the end which uniquely defines the constant. Attributes can be set for markers, lines, areas and character strings. Thus, there are four possible attribute arrays that are created using the four constants:
aAttrMarker := Array( GRA_AM_COUNT ) // attribute array for markers
aAttrLine := Array( GRA_AL_COUNT ) // attribute array for lines
aAttrArea := Array( GRA_AA_COUNT ) // attribute array for areas
aAttrString := Array( GRA_AS_COUNT ) // attribute array for string
The letters AM, AL, AA and AS are abbreviations for "attribute marker", "attribute line", "attribute area" and "attribute string". Each element of an attribute array defines a specific attribute. An element is identified by a #define constant which is prefixed by the attribute array prefix such as GRA_AM_, GRA_AL_, GRA_AA_ or GRA_AS_. An attribute is set by assigning a value to the appropriate element in the attribute array. For each attribute there is only a limited number of valid values which are again used in the form of #define constants.
aAttribute[ GRA_AL_TYPE ] := GRA_LINETYPE_SHORTDASH
In this line of code, the attribute "line type" receives the value for a short dashed line. Valid values for line types (more precisely, for the attribute GRA_AL_TYPE) are constants that are prefixed with GRA_LINETYPE_. For each attribute there is a group of #define constants like this that can be assigned as the value for the attribute.
Attributes are defined for a presentation space and therefore the attribute array must be passed to the presentation space after the attribute is inserted into the array. Only then are the attributes actually set and used for the graphic primitives during drawing. The functions used to assign the attribute arrays to the presentation space are listed in the following table.
Function | Description |
---|---|
GraSetAttrMarker() | Sets attributes for markers |
GraSetAttrLine() | Sets attributes for lines |
GraSetAttrArea() | Sets attributes for areas |
GraSetAttrString() | Sets attributes for character strings |
GraSetColor() | Sets colors for all graphic primitives |
GraSetFont() | Sets font for graphic output of characters |
The GraSetAttr..() functions pass an attribute array to a presentation space.
The attributes set for markers are used by the graphic primitive GraMarker(). The attributes for lines are used by GraLine() and GraSpline(). They are also used by GraArc() and GraBox() when a circle or a rectangle is drawn with a border (the border is a line). If a circle or a rectangle is to be filled with a pattern, the attributes for areas are used. These are defined by GraSetAttrArea(). The graphic primitives GraArc() and GraBox() use line attributes as well as area attributes. Attributes for character strings are used exclusively by GraStringAt(). The following tables give an overview of the attributes that can be set for markers, lines, areas and character strings.
Array element | #define | value | Description |
---|---|---|
GRA_AM_COLOR | GRA_CLR_* | Foreground color |
GRA_AM_BACKCOLOR | GRA_CLR_* | Background color |
GRA_AM_MIXMODE | GRA_FGMIX_* | Mix attribute for foreground color |
GRA_AM_BGMIXMODE | GRA_BGMIX_* | Mix attribute for background color |
GRA_AM_SYMBOL | GRA_MARKSYM_* | Marker symbol |
GRA_AM_BOX | {nXsize, nYsize} | Size of the marker symbol |
Array element | #define group | Description |
---|---|---|
GRA_AL_COLOR | GRA_CLR_* | Foreground color |
GRA_AL_MIXMODE | GRA_FGMIX_* | Mix attribute for foreground color |
GRA_AL_WIDTH | GRA_LINEWIDTH_* | Line width |
GRA_AL_TYPE | GRA_LINETYPE_* | Line type |
Array element | #define | value | Description |
---|---|---|
GRA_AA_COLOR | GRA_CLR_* | Foreground color |
GRA_AA_BACKCOLOR | GRA_CLR_* | Background color |
GRA_AA_MIXMODE | GRA_FGMIX_* | Mix attribute for foreground color |
GRA_AA_BGMIXMODE | GRA_BGMIX_* | Mix attribute for background color |
GRA_AA_SYMBOL | GRA_SYM_* | Fill pattern for areas |
Array element | #define | value | Description |
---|---|---|
GRA_AS_COLOR | GRA_CLR_* | Foreground color |
GRA_AS_BACKCOLOR | GRA_CLR_* | Background color |
GRA_AS_MIXMODE | GRA_FGMIX_* | Mix attribute for foreground color |
GRA_AS_BGMIXMODE | GRA_BGMIX_* | Mix attribute for background color |
GRA_AS_BOX | {nXsize,nYsize} | Size of each character |
GRA_AS_ANGLE | {nX,nY} | Output angle of character strings |
GRA_AS_SHEAR | {nX,nY} | Shear of characters (italics) |
GRA_AS_DIRECTION | GRA_CHDIRN_* | Write direction |
GRA_AS_HORIZALIGN | GRA_HALIGN_* | Horizontal alignment |
GRA_AS_VERTALIGN | GRA_VALIGN_* | Vertical alignment |
GRA_AS_EXTRA | nExtra | Distance between characters in character strings |
GRA_AS_BREAK_EXTRA | nBreakExtra | Distance between words |
Colors for graphic primitives
The function GraSetColor() defines the foreground and background color for the graphic primitives of the GRA engine. Colors defined using the function SetColor() are valid only for display in text mode and in hybrid mode. The GraSetAttr..() functions can individually set colors for markers, lines, areas and character strings that are then used instead of the globally defined colors. With the exception of lines, graphic primitives have a foreground and a background color. Lines have only a foreground color. The background color is not generally visible. It is used when displaying characters, which are always surrounded by a rectangle. The rectangle surrounding each character is drawn in the background color and the character itself (the letter) is displayed in the foreground color. The mix attribute for the background color must be defined in order to make the background color visible. Color mix attributes can be defined for the foreground color as well as for the background color. They determine how the colors of graphic primitives are mixed with each other when the output of a graphic primitive covers an already existing drawing.
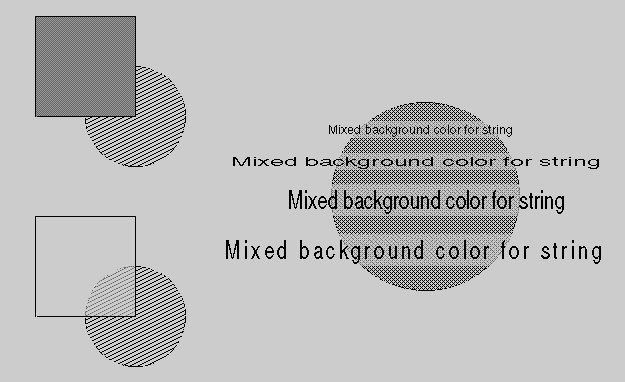
In this illustration, the circles are drawn first. The mix attribute for the foreground color is changed from GRA_FGMIX_OVERPAINT (the default value, that causes the new primitive to cover the old) to GRA_FGMIX_OR prior to display of the lower square. When this mix attribute is set, the foreground color of the square is mixed with the foreground color of the existing drawing. When GRA_FGMIX_OR is set, the mix occurs using a bitwise OR between the color values of the existing colors and the colors of the new graphic being drawn. The area where the circle and square are combined is visible in the mix color and this section appears somewhat lighter than the rest of the circle. The color of the rest of the square in the illustration is the result of the color mix between dark gray (square) and pale gray (window color).
In the right part of the illustration, character strings are drawn over a circle. Within the circle, the background color for characters is visible. It causes the fill pattern of the circle to appear lighter. In this case, the mix color is a result of the value GRA_BGMIX_OR for the mix attribute of the background color of GraStringAt(). By default the mix attributes are set so that the foreground color paints over everything already displayed (GRA_FGMIX_OVERPAINT) and the background color remains unaffected (GRA_BGMIX_LEAVEALONE). The mix attribute for the foreground color is always significant when graphic primitives overlap each other and the shared section is to remain visible. When character strings are displayed, the mix attribute for the background color should also be considered, since both the foreground color (letter) and the background color (the rectangle which surrounds a letter) are shown.
A special value for the color mix attribute for foreground colors is GRA_FGMIX_XOR. The result of displaying graphic primitives when this value is set is that the graphic primitives are deleted from the screen if they are drawn at the same location a second time. Also, the resulting mix color of a graphic primitive which paints over an existing drawing depends on the color of the existing drawing and the color of the graphic primitive. The following two tables list the constants that can be used for the mix attribute of the foreground color and the background color:
Constant | Description |
---|---|
GRA_FGMIX_OVERPAINT | Paints over existing colors |
GRA_FGMIX_LEAVEALONE | Uses existing colors |
GRA_FGMIX_OR | Mixes new and existing colors using bitwise OR of the color bits |
GRA_FGMIX_XOR | Mixes new and existing colors using bitwise XOR of the color bits |
GRA_FGMIX_AND | Mixes new and existing colors using bitwise AND of the color bits |
GRA_FGMIX_NOTMERGESRC | Inverts mix color of GRA_FGMIX_OR |
GRA_FGMIX_NOTMASKSRC | Inverts mix color of GRA_FGMIX_AND |
GRA_FGMIX_NOTXORSRC | Inverts mix color of GRA_FGMIX_XOR |
GRA_FGMIX_INVERT | Inverts existing color |
GRA_FGMIX_NOTCOPYSRC | Inverts new color |
GRA_FGMIX_SUBTRACT | Inverts new color and mixes it with existing color using bitwise AND of the color bits |
GRA_FGMIX_MERGENOTSRC | Inverts new color and mixes it with existing color using bitwise OR of the color bits |
GRA_FGMIX_MASKSRCNOT | Inverts existing color and mixes it with new color using bitwise AND of the color bits |
GRA_FGMIX_MERGESRCNOT | Inverts existing color and mixes it with new color using bitwise OR of the color bits |
GRA_FGMIX_ZERO | Resulting color is black (all color bits are set to 0) |
GRA_FGMIX_ONE | Resulting color is white (all color bits are set to 1) |
Constant | Description |
---|---|
GRA_BGMIX_OVERPAINT | Paints over existing colors |
GRA_BGMIX_LEAVEALONE | Uses existing colors |
GRA_BGMIX_OR | Mixes new and existing color using bitwise OR of the color bits |
GRA_BGMIX_XOR | Mixes new and existing color using bitwise XOR of the color bits |
Set font for characters
The function GraSetFont(), along with GraSetAttrString(), affects the display of characters and character strings. GraSetFont() passes to a presentation space an XbpFont object defining the font for graphic display of characters. By default, the font "System VIO" is used. To change the font, an XbpFont object must be created and passed to the presentation space. The size can be defined when an XbpFont object is created (the size is in points). When an XbpFont object is created using XbpFont():new(), a font is not yet available, but is only loaded into memory by the method :create() (refer to the section "Basics of Xbase Parts" and the discussion of their life cycle). Also, an XbpFont object can only load fonts that are available as system fonts. A distinction must be made between fonts that are device dependent (for example, fonts only available for a printer) and those that are device independent.
It is recommended that fonts be loaded using XbpFont():new() and then modifid using GraSetAttrString(). The attributes for character strings allow the creation of diverse font displays. This begins with the size of individual characters and includes the angle in which a character is displayed relative to the horizontal axis.
Because of internal differences between XbpFont()and GraSetAttrString(), XbpFont() should be used only to load a font and GraSetAttrString() should be used to define the attributes for displaying characters. It should be noted that many attributes are valid only for vector fonts. A vector font is drawn as the outline of the letters using graphic primitives and then the outline is filled. A letter in a bitmap font consists of a raster image. Because of this, the characters of a bitmap font cannot be automatically converted to italics. In order to display the characters of a bitmap font in italics, a separate italic bitmap font is required.
If you see anything in the documentation that is not correct, does not match your experience with the particular feature or requires further clarification, please use this form to report a documentation issue.