Statement VAR Foundation
Declaration of instance variables (member variables of instances of a class).
VAR <VarName,...> ;
[READONLY] [ASSIGNMENT HIDDEN | PROTECTED | EXPORTED] [NOSAVE]
or
VAR <MessageName> [IS <VarName>] [IN <SuperClass>] ;
[READONLY] [ASSIGNMENT HIDDEN | PROTECTED | EXPORTED] [NOSAVE]
The statement VAR is used within the class declaration (between the CLASS...ENDCLASS statements). It declares a member variable as an instance variable. An instance variable is a member variable of instances of a class. All instances (objects) of a class have a separate set of the instance variables with the variables for each instance containing independent values. Note that an instance variable differs from a class variable which exists only once per class and whose value is the same for all objects of a class. When an instance object receives the message for a class variable, it forwards the message on to the class object.
With <MessageName> the message for accessing an instance variable can be defined. It represents an alternative name for the instance variable. If a <MessageName is defined, it must be used to access the variable <VarName>.
<MessageName> can be included when the instance variable does not exist in the declared class, but in one of its superclasses. When two or more superclasses exist which have the same names for instance variables, it is recommended that unique alternative names be defined for accessing the instance variables of each superclass. In this case the declaration is used in the form CLASS VAR..IS..IN.
The visibility of an instance variable is determined by the visibility attribute set with one of the statements EXPORTED:, PROTECTED: or HIDDEN: (see the section on each visbility attribute for more information). Write access is limited by the options READONLY or ASSIGNMENT independent of the visibility of an instance variable. The following table shows the meaningful combinations between visibility and assignment:
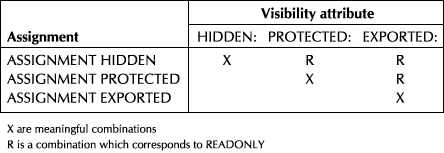
// The example illustrates the general procedure for the
// declaration and the initialization of instance variables.
CLASS classA // Class declaration
EXPORTED:
VAR varA1, varA2
METHOD init // Declares method :init()
ENDCLASS
METHOD init( n1, n2 ) // Program the method
::varA1 := n1 // Assigns beginning values
::varA2 := n2 // to the instance variables
RETURN self
If you see anything in the documentation that is not correct, does not match your experience with the particular feature or requires further clarification, please use this form to report a documentation issue.