Statement CLASS VAR Foundation
Declaration of class variables (variables of the class object)
CLASS VAR <VarName,...> ;
[SHARED] [READONLY] [ASSIGNMENT HIDDEN | PROTECTED | EXPORTED]
or
CLASS VAR <MessageName> [IS <VarName>] [IN <SuperClass>] ;
[SHARED] [READONLY] [ASSIGNMENT HIDDEN | PROTECTED | EXPORTED]
The declaration CLASS VAR is used within the class declaration (between the CLASS...ENDCLASS statements). It declares a member variable as a class variable. A class variable is a member variable of the class object and it exists only once per class. Its value is the same for all instances of the class. When an instance of the class (an object) receives the message for a class variable, it forwards the message on to a class object.
With <MessageName> the message (or name) used for accessing a class variable can be changed. It defines an alternative name for a class variable. If a <MessageName> is defined, the variable <VarName> can only be accessed using <MessageName>.
<MessageName> can be defined when a class variable exists only in one of the superclasses to the class incorporating <MessageName>. When two or more superclasses exist which have the same names for class variables it is recommended that alternative names be declared for accessing class variables in each superclass. In this case the declaration is used in the form CLASS VAR..IS..IN.
The visibility of a class variable is determined by the visibility attribute set with one of the keywords EXPORTED:, PROTECTED: or HIDDEN: (see the section on each visibility attribute for more information). Write access that is specified by the options READONLY or ASSIGNMENT is not dependent on the visibility of a class variable. The following table contains the meaningful combinations between visibility attributes and data assignment:
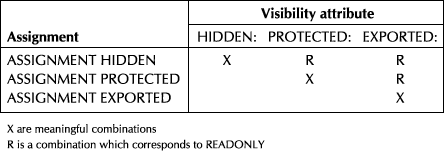
If a class variable is declared with the option SHARED, the value or contents of this class variable is also used in future subclasses. In this case, the class variable exists only in the declared class and its contents are accessable by all future subclasses (the value is the same). Without the option SHARED, each subclass receives its own copy of the class variable and accesses its own value for the class variable (see declaration CLASS).
// The example illustrates the procedure for the initialization
// of class variables. The class method :initClass() is
// first declared and then programmed.
CLASS Window
CLASS VAR Stack
CLASS METHOD initClass // Declares class method
EXPORTED:
VAR nTop, nLeft, nBottom, nRight
METHOD Hide, Show, Move, Resize, Close
ENDCLASS
CLASS METHOD Window:initClass // Code for class method
::Stack := {} // Initializes
RETURN self // class variables
If you see anything in the documentation that is not correct, does not match your experience with the particular feature or requires further clarification, please use this form to report a documentation issue.