Building WAP sites Professional
The major difference between WAP applications and Web applications is the mark-up language required for the presentation of content. HTML (Hyper Text Markup Language) is the mark-up language for the Web, while WML (Wireless Markup Language) is the one for WAP devices. WML is easy to learn and there are some very good tutorials and crash courses available in the Internet that give you a complete picture about this language in almost no time (refer to WAP and WML related online resources). Therefore, we are not going to explain WML in depth but will concentrate on some major WML topics and what else must be known to build a WAP site.
The most important part to understand in WML is that one WML document may contain several pages. This is different to HTML where one HTML file contains one page to be displayed in a browser. A WML file, in contrast, contains so called "cards" (pages) which make up a "card-deck" (document). This is pretty comfortable compared to HTML since several pages can be combined in one WML file. In addition, WML allows a programmer to define the sequence of card display, or how a user may navigate through a card-deck.
Before you start thinking now "Great, I can put an entire WAP site into one file", be warned: you can't, unless it is a small one. The reason is that you are dealing with WAP devices that have a limited amount of memory and may support only a limited transfer rate of 9600 baud. Another limitation is that WAP devices don't come with a 17 inch monitor supporting a 1280x1024 resolution. Instead, a WAP developer deals with something like modest 90x65 pixel LCD screens that fit into a mobile phone and may not be able to display more than four lines of text without scrolling.
It is the limitation of the hardware that caused the language designers to introduce the card/card-deck functionality into WML and it makes real good sense. Just imagine how much information can be transfered with 5 kB of data. This amount can be transfered in reasonable time at a 9600 baud transmission rate and it can include more text than you have read so far from the beginning of this chapter. As a matter of fact, the first five paragraphs of this chapter make up 2782 bytes (white space included).
If you were to include these five paragraphs in a WAP site, you could organize them in one WML file and use a card for each paragraph. The WML file (including mark-up) would not exceed 5 kB in size so that it could be transfered entirely to a mobile phone in a reasonable time. The user could then display the text by skipping through the card-deck and scrolling the contents of each card in the LCD display without the need to connect to a WAP provider each time the next paragraph, or card, is requested.
However, it would not be a good idea to include so much text in a card that is to be displayed in a mobile phone. In contrast, if the WAP device was a PDA (Personal Digital Assistant, hand held computer) the text of one paragraph could fit into the display and the one paragraph/one card approach could be appropriate.
This introductory discussion should make you aware of a variety of issues that must be taken into consideration when planning and designing WAP sites. "What are the hardware limitations of the WAP device?" and "How much text can be displayed?" are the most important questions to be answered. The most restrictive device in this context is a mobile phone and that's why we are using it as the candidate for discussing basic WML principles.
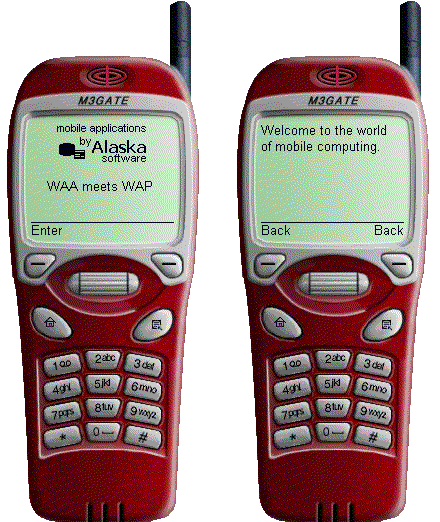
These illustrations are screen shots of the M3Gate WAP browser that emulates a mobile phone not only visually but also in user interaction. It is a great tool for testing a WAP site during development without a WAP phone since M3Gate exposes all characteristics of a mobile phone (Note: M3Gate can also be run in PDA mode). The two phones display a WML document consisting of two cards, and this is the WML code used to produce the screen shots:
01: <?xml version="1.0"?>
02: <!DOCTYPE wml PUBLIC "-//WAPFORUM//DTD WML 1.1//EN"
03: "http://www.wapforum.org/DTD/wml_1.1.xml">
04: <wml>
05: <card id="card1" newcontext="true">
06: <do type="accept" label="Enter">
07: <go href="#card2"/>
08: </do>
09:
10: <p align="center">
11: <img src="alaska.wbmp" alt="Alaska Software"/>
12: </p>
13:
14: <p align="center"><br/>
15: WAA meets WAP
16: </p>
17: </card>
18:
19: <card id="card2">
20: <do type="accept" label="Back">
21: <go href="#card1"/>
22: </do>
23: <p>
24: Welcome to the world
25: of mobile computing.
26: </p>
27: </card>
28: </wml>
WML is implemented in XML (eXtensible Markup Language) and that's why each WML file must begin with the code shown in lines #1-3. However, this is just informational and nothing you are bothered with when using the WML classes of WAA. The code is structured by tags -just as in HTML- and begins/ends always with <wml> .. </wml>.
The characteristical WML tags are <card> (lines #5 and #19) and the <do><go></go></do> tags. The former separate the contents of individual cards in the card-deck while the latter define navigational tasks, which is unknown in HTML. A task is executed when the user activates a label being displayed in the WAP device, and the document specified in the "href=" attribute of the <go> tag is loaded/displayed as a result.
This is about all you need to know at the beginning about WML and we look now into the PRG source code you would use in a WAA package DLL to create the same result:
01: FUNCTION MyWMLFunc( oWMLDeck, oContext )
02: LOCAL oWMLCard := oWMLDeck:newCard()
03:
04: oWMLCard:put( '<p>' )
05: oWMLCard:img( 'alaska.wbmp', 'Alaska Software' )
06: oWMLCard:put( '</p>' )
07: oWMLCard:paragraph( '</br>WAA meets WAP', 'center' )
08:
09: oWMLCard := oDeck:newCard()
10: oWMLCard:paragraph( 'Welcome to the world of mobile computing.' )
11: RETURN .T.
This function gets passed from the WAA a WMLDeck object which represents a WML card-deck. The individual cards are created by :newCard() which returns a new WMLCard object. Contents and attributes for WML tags are then defined using methods of WMLCard objects. That's all about WML class usage. The required WML header is built into the classes as well as default tasks for navigating through a card-deck.
The only thing left to know is how to instruct the WAA to instantiate the WMLDeck class and pass the object to your DLL. This is easiest been done by this PRG code:
01: #pragma Library( "WAA1WAP.LIB" )
02:
03: PROCEDURE Main
04: LOCAL oWMLDeck := WMLDeck():new()
05: LOCAL oWMLCard := oWMLDeck:newCard()
06:
07: oWMLCard:setWaa( "MyDLL", "MyWMLFunc" )
08: oWMLCard:setTimeOut( 0, oWMLCard:getWAALink() )
09:
10: SET ALTERNATE TO Index.wml
11: SET ALTERNATE ON
12:
13: ? oWMLDeck:toWML()
14: RETURN
The code's sole purpose is to create the initial WML file that is returned by a HTTP server to a WAP device when a user starts visiting a WAP site. It creates the file Index.wml which serves as the entry point into a WAA controlled WAP site. The name of a package DLL and the function to invoke are defined in line #7. Both are required for the :getWaaLink()method in line #8. This method simply returns a character string containing the link required for accessing the WAA. The link is passed to the method:setTimeOut(), which, in turn, creates the WML code for a timer. When the time-out period is reached, the WAP device loads automatically the WML document specified with the link. Since the time-out value is set to 0, the DLL function defined in line #7 is immediately invoked when Index.wml is loaded into a WAP device.
The resulting Index.wml file must then be stored in the HTTP server's document root directory. It contains this WML code:
01: <?xml version="1.0"?>
02: <!DOCTYPE wml PUBLIC "-//WAPFORUM//DTD WML 1.1//EN"
03: "http://www.wapforum.org/DTD/wml_1.1.xml">
04: <wml>
05: <card id="card1" ontimer="/cgi-bin/waa1gate.exe?
06: WAA_HTML3CLASS=WMLDeck&
07: WAA_PACKAGE=MyDll&
08: WAA_FORM=MyWMLFunc">
09: <timer value="0"/>
10: </card>
11: </wml>
The class to be instantiated by the WAA server is encoded in the CGI string which is returned to the HTTP server when the time-out period is reached. The CGI variable WAA_HTML3CLASS holds the name of that class.
If you see anything in the documentation that is not correct, does not match your experience with the particular feature or requires further clarification, please use this form to report a documentation issue.