Introduction Foundation
The concept of an object can be illustrated using an example from the real world. Imagine two objects which have different colors that can both travel. These objects have the two essential aspects necessary for describing objects either in the real world or in relation to OOP: objects have state and behavior.
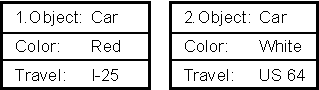
The two objects have different state but share the same behavior. One could expand the example by classifying everything that has a color and can travel like a car. This leads to the concept of a class. A class is an abstraction of things from the real world and describes the state and behavior of all objects that can be classified using a particular definition. The two car objects above have the state and the behavior described by the class car. Below is a generalized description of the car class:
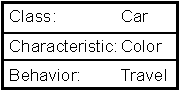
Class and object are two of the most important concepts in object-oriented programming. The class defines the state and the behavior which describe the class. Objects of a class are generated based on this definition. The process of creating objects from the class definition is referred to as creating an instance. The term instance is frequently used and is synonymous with the term object. A car object is an instance of the car class.
The example can be expanded to show other important OOP concepts by adding the abstraction of a bicycle as an object. Consider adding two bicycles: a red one and a white one. Now there are four objects, each of which has a color and can travel. Two of these objects are white and two are red.
Should the bicycle be an object of the car class (or vice versa), just because cars and bicycles have the same state (color) and display the same behavior (travel)? Other than these specific aspects, the two have little in common. They are started differently, are powered by different sources, turn differently, etc. Yet both objects have state and behavior which allow a common definition. The car and bicycle objects could all be defined as vehicles, with each vehicle having the state colorand displaying the behavior travel. In this sense, the "travel" behavior is similar because both vehicles have wheels and both travel on roads.
If this classification scheme is transferred to OOP, a vehicleclass can be defined that describes all the state and behavior of objects with wheels that travel on roads.
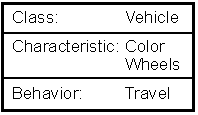
In order to describe a car object or a bicycle object it is no longer necessary to say they are objects which have a color and wheels and that can travel. This is all implicit if they are described as vehicles. Within this group of vehicles there are differences: one type of object has a motor and the other does not, for example. To define classes for bicycles and cars it is sufficient to define the state and behavior which are specific to a bicycle and a car. The other state and behavior can just be taken from the vehicle class. In OOP the vehicle class is called the superclass, since it describes things that are common to many classes. The car and bicycle classes are derived from this superclass.
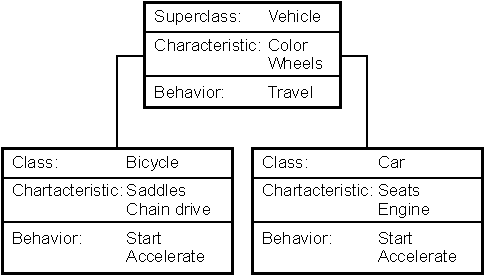
The behavior and state which is common between a bicycle object and a car object is described in the vehicle class. The vehicle class is the superclass for the bicycle class and the car class. The bicycle and car classes are called subclasses, because they assume the state and behavior of another class. This is called inheritance in OOP, and implies that the travel behavior does not need to be defined in the bicycle and car classes. When a bicycle object is created using the bicycle class, it automatically receives the state and behavior defined for the vehicle class as well. This is also true when a car object is created from the car class: a car object "inherits" the behavior travel from the vehicle class.
This process of inheritance plays a central role in OOP. In describing objects, a very general class is first created and this class is specialized using additional subclasses. A car object and a bicycle object can be schematically represented as follows:
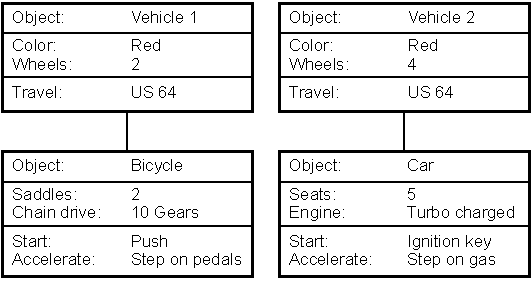
This abstraction of a car and a bicycle can be used to describe a real world situation:
Two red vehicles are on US Highway 64. One must be a car because it is a vehicle that has four wheels. The other might be either a bicycle or a motorcycle because it has two wheels. A closer look shows that one vehicle is a five passenger car with a turbo charged engine and the other vehicle is a tandem bicycle with 10 gears. The bicycle and car objects are first described as objects of the superclass vehicle but this does not adequately describe or differentiate the two vehicles. A closer look at the objects shows additional details about each vehicle. Without the vehicle object the color, number of wheels and road being traveled are not defined for each car and bicycle. The vehicle-car object and vehicle-bicycle object are units used to create a precise description of the objects.
In the class definitions shown above, both the bicycle and car classes can start and accelerate. Why is this behavior not defined in the vehicle class? What is the difference between travel and accelerate? The process of traveling is the same for a bicycle and a car (wheels turn on a road) but the acceleration and method of starting are different. Only the name and the effect is the same. How the object's behavior is actually carried out can be completely different in the two classes. A bicycle accelerates by someone pushing hard on the pedals while the same effect is achieved in a car by simply stepping on the gas pedal.
This situation is enormously important in OOP. The behavior of two objects is described using the same name, but the implementation of the behavior is different. In OOP this is called polymorphism which literally means "many forms".
Almost all concepts in OOP are derived from the real world and attempt to abstract the real world. There is the concept of an object, which essentially unites the state and behavior of things. The class gives a general definition of objects by specifying what state and behavior each object has. Objects and classes are inseparably linked with each other: without a class there is no object. An object is always the instance of a class.
The relationship between superclasses and subclasses is extremely important in describing classes. A superclass contains a general definition and the subclass implements specialization. When a class is based on one or more other classes, the resulting class hierarchy is often referred to as the inheritance tree.
When an object is created from a subclass, it always inherits the state and the behavior defined in the superclass as well as the subclass. Objects can be created from subclasses that differ from the superclass in only a few ways.
When a behavior has the same name and results in the same basic effect (but can be implemented differently) in various classes, the situation is referred to as polymorphism.
The terminology used in object-oriented programming (OOP) is not consistent and often leads to confusion when programmers make the transition from procedural programming to OOP. Specific terms are used in different levels of abstraction, often synonymously. The result is that the programmer's basic understanding of the term object and the power of OOP is generally slow to develop.
At a basic level, an object is a data type with a complex structure that unites values (state) and functionality (behavior). Each object uses its own variables. The variables of an object are called member variables to distinguish them from the normal memory variables used in procedural programming. Memory variables can contain objects that in turn have their own variables (the member variables) with their own variable names.
Along with variables (state), an object also has its own functions (behavior). The term method is used for the functions that are part of an object in order to distinguish them from traditional procedural functions. Methods can only be called or executed by an object. They contain program code and are similar in other ways to procedures or user-defined functions. When an object executes a method, the object is always visible within the code of the method.
Class is the term used to describe an abstract definition of a data type which is called an object. The class defines the structure of its objects by specifying the member variables and methods of each object of the class. Within a program the class is defined in the class declaration. The class declaration declares the member variables and methods that objects of the class can use during runtime of the application.
The compiler creates the class function from the class declaration. The class function returns the class object which represents the class at runtime. The class object exists only once per class and only the class object can create instances (or objects) of the class.
The class object can have its own member variables and its own methods: class variables and class methods. A variable declared as a class variable exists only once per class and is similar to a file-wide STATIC variable in procedural programming. A class method can be executed only by the class object.
The following diagram illustrates the terms which are used in the Xbase++ documentation and shows the relationships between them:
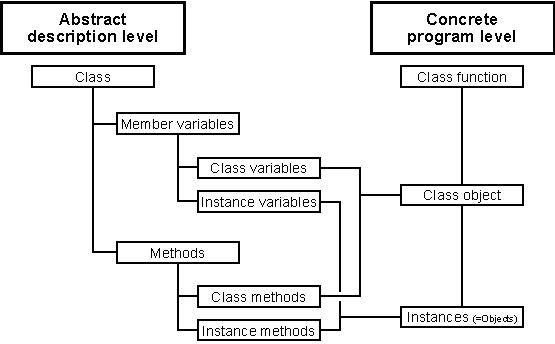
The diagram displays the class as an abstract definition describing the structure of objects in terms of their member variables and methods. A class is defined in the class declaration, which is translated by the compiler to the class function. The class function creates the class object at runtime. Only the class object can create instances of the class.
The term instance refers to an object created by the class object at runtime. Instances as well as class objects are of the object data type, since they can have member variables and methods.
The member variables of the instances are also called instance variables. All instances have the same set of instance variables but may contain different values in them. Class variables exist only in the class object but can be read and/or changed by any instance, since all instances can access "their" class object. Since the class object exists only once, the values in the class variables are the same regardless of which instance is used to access them.
The term method is used to refer to both instance methods and class methods. Instance methods are methods which can be executed only by instances. Class methods can be called by the class object or by instances, but they are only executed by the class object. The term instance and object are used synonymously in Xbase++ documentation.
If you see anything in the documentation that is not correct, does not match your experience with the particular feature or requires further clarification, please use this form to report a documentation issue.