My first Web application Professional
This is a discussion of a small Web application project to provide you with the very basic concepts to understand when using WAA1. We will build an HTML page with an entry field where a user can enter a name. The name is sent to the Web application where it is processed and a response is sent back to the Web browser.
Let us first have a look at the Web browser, or client side, of the application. It consists of one HTML file which looks like this when displayed with a Web browser:
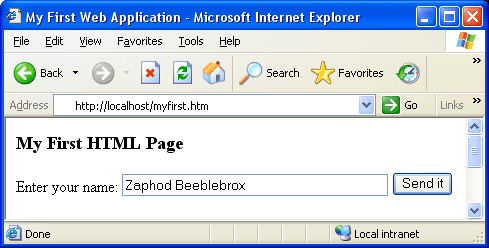
The user is prompted to enter his name in an entry field and a pushbutton is displayed. The HTML code for this page consists of only 16 lines:
01: <HTML>
02: <HEAD>
03: <TITLE>My First Web application</TITLE>
04: </HEAD>
05: <BODY>
06: <B><FONT SIZE=+1>My First HTML Page</FONT></B><BR>
07:
08: <FORM ACTION="/cgi-bin/waa1gate.exe" METHOD=post>
09: <INPUT TYPE=hidden NAME="WAA_PACKAGE" VALUE="myfirst">
10: <INPUT TYPE=hidden NAME="WAA_FORM" VALUE="SayHello">
11: Enter your name: <INPUT TYPE="text" NAME="TheName" SIZE=20>
12: <INPUT TYPE=submit VALUE="Send it"> <P>
13: </FORM>
14:
15: </BODY>
16: </HTML>
Note for WAA version 1.90.347 and higher: This sample utilizes the myfirst.dll WAA package included in the WAA Server sample collection. Upon WAA version 1.90.347 and higher the samples in general no longer require static html pages in the root directory of the WWW server, except the index page of course. In order to be able to follow-through upon explanations in this chapter, first create the html page myfirst.htm on the root directory of the web Server. Then this page can be viewed in a web browser when the URL http://<servername>/myfirst.htm is entered.
If a different WAA gateway than the waa1gate.exe shall be used, the FORM ACTION in the html code above needs to be modified accordingly.
The interesting part of the code is enclosed in the <FORM> and </FORM> tags. In line 8, the ACTION= attribute instructs the HTTP server to invoke the gateway WAA1GATE.EXE and pass the INPUT values on to it when the form is submitted (by clicking the "Send It" pushbutton). Two of the input values are defined as hidden (lines 9 and 10). They are not displayed in the browser, but have a name and a value. You will program such lines frequently since "WAA_PACKAGE" indicates the package DLL the WAA server is to use and "WAA_FORM" defines the function in the package DLL which creates a response.
The gateway receives information from the HTTP server and forwards it on to the WAA server. There, the file MYFIRST.DLL is actually addressed when the pushbutton "Send it" is clicked. In the DLL, the function SayHello() is programmed and processes the entered string.
The response of the Web application is displayed when the user clicks the pushbutton. This way, the entered string is submitted to the HTTP server together with the hidden values. The result is this:
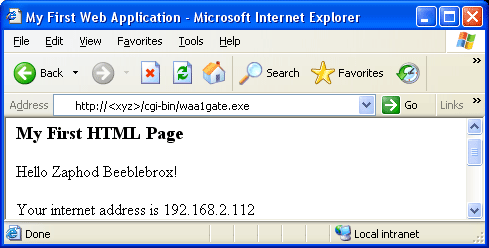
Since the response is displayed in the Web browser, it is clear that the Web application (or package DLL) assembles an HTML page and returns it via the gateway to the HTTP server which, in turn, forwards it to the Web browser, or client station. The assembly of the HTML page is done with the following 24 lines of Xbase++ code which represent the entire Web application:
01: FUNCTION _Register( oPackage )
02: oPackage:registerForm( "SAYHELLO" )
03: RETURN .T.
04:
05: FUNCTION _Version()
06: RETURN "Version 1.0"
07:
08: FUNCTION _Copyright()
09: RETURN "Alaska Software, (c) 1998. All rights reserved."
10:
11: FUNCTION SayHello( oHTML, oContext )
12: LOCAL cName := oHTML:getVar( "TheName" )
13: LOCAL cINet := oContext:getRemoteAddr()
14:
15: IF Empty( cName )
16: oHTML:message( "Error in SayHello()", "No name specified" )
17: ELSE
18: oHTML:header()
19: oHTML:put( "<P>Hello " + cName + "! </P>" )
20: oHTML:put( "<P>Your internet address is " + cINet + "</P>" )
21: oHTML:footer()
22: ENDIF
23:
24: RETURN .T.
The code starts with the declaration of three compulsory functions that must exist in each Web application: _Register(), _Copyright() and _Version(). The _Register() function receives a package object from the WAA server and registers all function names that may be called from an HTML page. This is accomplished by passing the function names as character strings to the :registerForm() method of the object. Each registered function is responsible for the assembly of one HTML page, or Form. Have a look again at line 10 in the HTML file:
10: <INPUT TYPE=hidden NAME="WAA_FORM" VALUE="SayHello">
The name "WAA_FORM" of the hidden INPUT TYPE indicates a registered form-function in a package DLL, while VALUE= specifies the function's name. Therefore, the function SayHello() is invoked by the WAA server to process data received via the gateway.
For data processing, each registered form-function receives two objects: one represents the HTML page on the client side (oHTML) and the other one holds information about the context, or connection, when the function is called (oContext). The oHTML object knows what was entered in the entry field, and we know the name of the variable from line 11 in the HTML file:
11: Enter your name: <INPUT TYPE="text" NAME="TheName" SIZE=20>
By passing the variable name "TheName" to the :getVar() method of the oHTML object in line 12 of the PRG code, we retrieve its value, or what was entered by the user. From here on it is pretty simple to process the data and to assemble an HTML page as a response. It is done in the lines 18-21 by calling various methods of the oHTML object.
The important part is that the assembly of the HTML page is embedded within the method calls :header() and :footer(). These methods mark the begin and the end of an HTML page returned to the client station. Between :header() and :footer(), the method :put() is used in the example to create two paragraphs (HTML tags <P> and </P>) in the returned HTML page. Both paragraphs contain information retrieved from the oHTML and oContext object. The RETURN value .T. (true) of the function tells the WAA server that the HTML page is complete and can be returned to the HTTP server. If the return value would be .F. (false), the WAA server would not send the assembled page.
So far, we have seen the client side (HTML file) and the server side (PRG file) of the Web application. Now let's see how to put the pieces together. The HTML file must be located in a directory visible to the HTTP server. Usually it is a sub-directory of \<ROOT>\htdocs where <ROOT> is the root directory of the HTTP server.
The PRG file must be compiled and linked to a DLL file which can be loaded dynamically. The easiest way to accomplish this is to use a project file and let the Alaska ProjectBuilder (PBUILD.EXE) do the job. Assuming the example PRG code is contained in the file MYFIRST.PRG, the project file (PROJECT.XPJ) looks like this:
[PROJECT]
COMPILE = xpp /dll:dynamic /w /wi /wl /wu
COMPILE_FLAGS = -q
DEBUG = yes
GUI = no
LINKER = alink
LINK_FLAGS =
RC_COMPILE = arc
RC_FLAGS = -v
PROJECT.XPJ
[PROJECT.XPJ]
MYFIRST.DLL
[MYFIRST.DLL]
MYFIRST.PRG
Note the compiler switch /dll:dynamic. It must be used for creating a package DLL since the WAA server loads package DLLs dynamically. The result of the ProjectBuilder is the file MYFIRST.DLL which contains the Web application. All what is left to do is to edit the WAA1SRV.CFG file and add the name and location of the new Web application. For example, we add this line to the configuration file:
addpkg = \alaska\waa1w32\source\samples\waa\misc\myfirst
When WAA1SRV.EXE starts, it loads the file MYFIRST.DLL and is ready to respond to user input made in the HTML page on the client station.
Summary
This chapter has outlined the basic concepts for developing Web applications using the Alaska Web Application Adaptor.
If you see anything in the documentation that is not correct, does not match your experience with the particular feature or requires further clarification, please use this form to report a documentation issue.